The object of CENPyOlpCsvParserOperator class can be used in callback methods to communicate with .csv files.
[Example 1]
def PostProcessOperationAttributes(poa):
csvParser = poa.GetCsvParserOperator()
# Get the path to the TechTabs folder and CSV file:
# Do not forget to add "import inspect, os" at the top of the file
# currentPythonFilePath = os.path.abspath(inspect.getfile(inspect.currentframe()))
# scriptsDirectoryPath = os.path.dirname(currentPythonFilePath)
# parentDirectoryPath = os.path.dirname(scriptsDirectoryPath)
# csvFilePath = str(parentDirectoryPath + "\\TechTabs\\Test.csv")
# or simply:
csvFilePath = str(os.path.dirname(os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe())))) + "\\TechTabs\\Test.csv")
iRet = csvParser.LoadCsvFile(csvFilePath)
if iRet == 0:
logging.LogInfo("Successfully imported CSV file: " + str(csvFilePath))
csvParser.SetSeparator(",")
numberOfRows = csvParser.GetNumberOfRows()
numberOfColumns = csvParser.GetNumberOfColumns()
cell12 = csvParser.GetCell(1, 2)
cellB1 = csvParser.GetCellByColumnName('B', 2)
row1 = csvParser.GetRow(1)
row2 = csvParser.GetRow(2)
# expected result:
# numberOfRows = 4; numberOfColumns = 4, cell12 = 'a1', cellB1 = 'b1'
# row1 = ['A','b','c']
# row2 = ['a1','b1','c1']
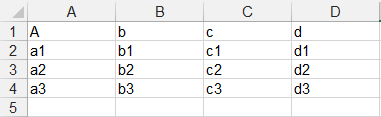
•
|
LoadCsvFile(filepath: string)
|
|
|
absolute path to the csv file
|
[Example]
# Get the path to the TechTabs folder and CSV file:
# Do not forget to add "import inspect, os" at the top of the file
# currentPythonFilePath = os.path.abspath(inspect.getfile(inspect.currentframe()))
# scriptsDirectoryPath = os.path.dirname(currentPythonFilePath)
# parentDirectoryPath = os.path.dirname(scriptsDirectoryPath)
# csvFilePath = str(parentDirectoryPath + "\\TechTabs\\Test.csv")
# or simply:
csvFilePath = str(os.path.dirname(os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe())))) + "\\TechTabs\\Test.csv")
iRet = csvParser.LoadCsvFile(csvFilePath)
if iRet == 0:
logging.LogInfo("Successfully imported CSV file: " + str(csvFilePath))
•
|
GetNumberOfRows() : Int
|
|
|
Number of rows in the table
|
[Example]
numberOfRows = csvParser.GetNumberOfRows()
•
|
GetNumberOfColumns() : Int
|
|
|
The number of columns of the file
|
[Example]
numberOfColumns = csvParser.GetNumberOfColumns()
•
|
GetCell(columnIndex: unsigned int, rowIndex: unsigned int) : string
|
|
|
The column-index.
|
|
|
The row-index.
|
|
|
Content of cell
|
The content of the table at column
[Example]
cell12 = csvParser.GetCell(1, 2)
•
|
GetCellByColumnName(columnName: string, rowIndex : unsigned int) : string
|
|
|
The column-name.
|
|
|
The row-index.
|
|
|
Content of cell
|
Accessing a cell by column-name(e.g. A, B, .., AA, AB,..) and a row-index.
[Example]
cellB1 = csvParser.GetCellByColumnName('B', 2)
•
|
SetSeparator(separator: string)
|
Setting the separator-character in the csv-file.
Remark: The default separator is given by ';'.
[Example]
csvParser.SetSeparator(",")
•
|
GetRow(rowIndex: unsigned int) : list
|
|
|
Text of the message
|
|
|
a list of cell values in the row
|
[Example]
row1 = csvParser.GetRow(1)
|